Let’s say you want to dynamically create modal popup in your Spring Boot project using Twitter Bootstrap 4 to show the user a ‘warning popup’ before deleting a data or edit the contents on the fly in same page. Not to mention I’ve used Thymeleaf as my template engine.
In this tutorial I’m gonna show how to achieve this.
I assume you have already included required Bootstrap resources and necessary jQuery library in your project and are ready to implement modal popup. Let’s copy the modal code from below:
<div class="modal modal-warning fade in" id="myModal">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span></button>
<h5 class="modal-title">Delete User</h5>
</div>
<div class="modal-body">
<h3>Are you sure want to delete this user?</h3>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-outline pull-left" data-dismiss="modal">Close</button>
<a type="button" class="btn btn-danger"><i class="fa fa-check"></i> Yes</a>
</div>
</div>
</div>
</div>
It’ll produce something like this:
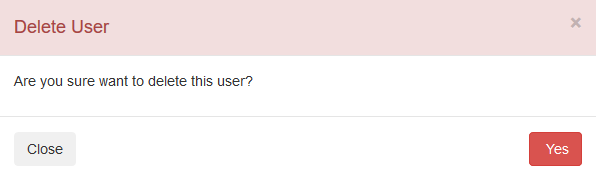
Now, the next step actually the final step. Make the modal dynamically generate for all the data in table.
I’ve some data in the table like below now I want to delete a user but before that I want that modal to generate dynamically for all data.
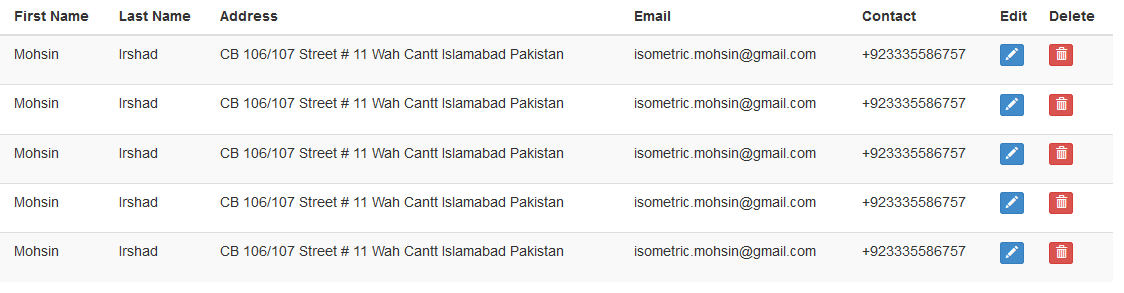
On the ‘Red’ delete button the popup will generate.
<a data-toggle="modal" data-target="#modal-warning" th:attr="data-target='#modal-warning'+${user.id }"><span class="glyphicon glyphicon-trash"></span></a>
Here “${user.id}” is my thymeleaf object.
Now, let’s make the modal dynamic with the id:
<div class="modal modal-warning fade in" th:id="modal-warning+${user.id }" >
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span></button>
<h5 class="modal-title">Delete User</h5>
</div>
<div class="modal-body">
<h3>Are you sure want to delete this user?</h3>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-outline pull-left" data-dismiss="modal">Close</button>
<a type="button" class="btn btn-outline" th:href="@{/user/delete/{id}(id=${user.id})}"><i class="fa fa-check"></i> Yes</a>
</div>
</div>
</div>
</div>
Here “th:href=”@{/user/delete/{id}(id=${user.id})}” is your POST URL.
You can also edit any data inside the modal by adding a form.
That’s all! We’re done!
If you’ve any confusion please let me know 🙂