Alright cloud ninjas, let’s be honest. Does anyone else feel like “cloud-native” has become the tech industry’s version of the latest fashion trend? Everywhere you turn, there’s another tool, another framework, another “must-have” for your cloud architecture. It’s enough to make your brain hurt.
Don’t get me wrong please, I’m all for innovation. But between the constant vendor hype and the ever-shifting trends, we engineers are left feeling like hamsters on a tech trend wheel. Is anyone else with me on this?
We’ve got senior devs (you know, the 7-10 year veterans) scratching their heads as the pendulum swings back from “cloud everything” to a mix-and-match approach. And new engineers fresh out of boot camp? They’re drowning in a sea of conflicting advice and confusing abbreviations.
Today’s cloud-native world is full with confusion, and it’s no wonder why. The sheer volume of tools and conflicting advice makes it hard to know where to turn. A quick Google search shows ten different answers to the same question, leaving engineers more bewildered than enlightened. The cloud-native space is evolving at breakneck speed. We crave more, faster, reflecting modern consumerism’s insatiable appetite. This rapid development pace, combined with a plethora of vendors, complicates matters further.
To illustrate how many of us feel, here’s a popular meme that perfectly captures the current state of cloud-native development:
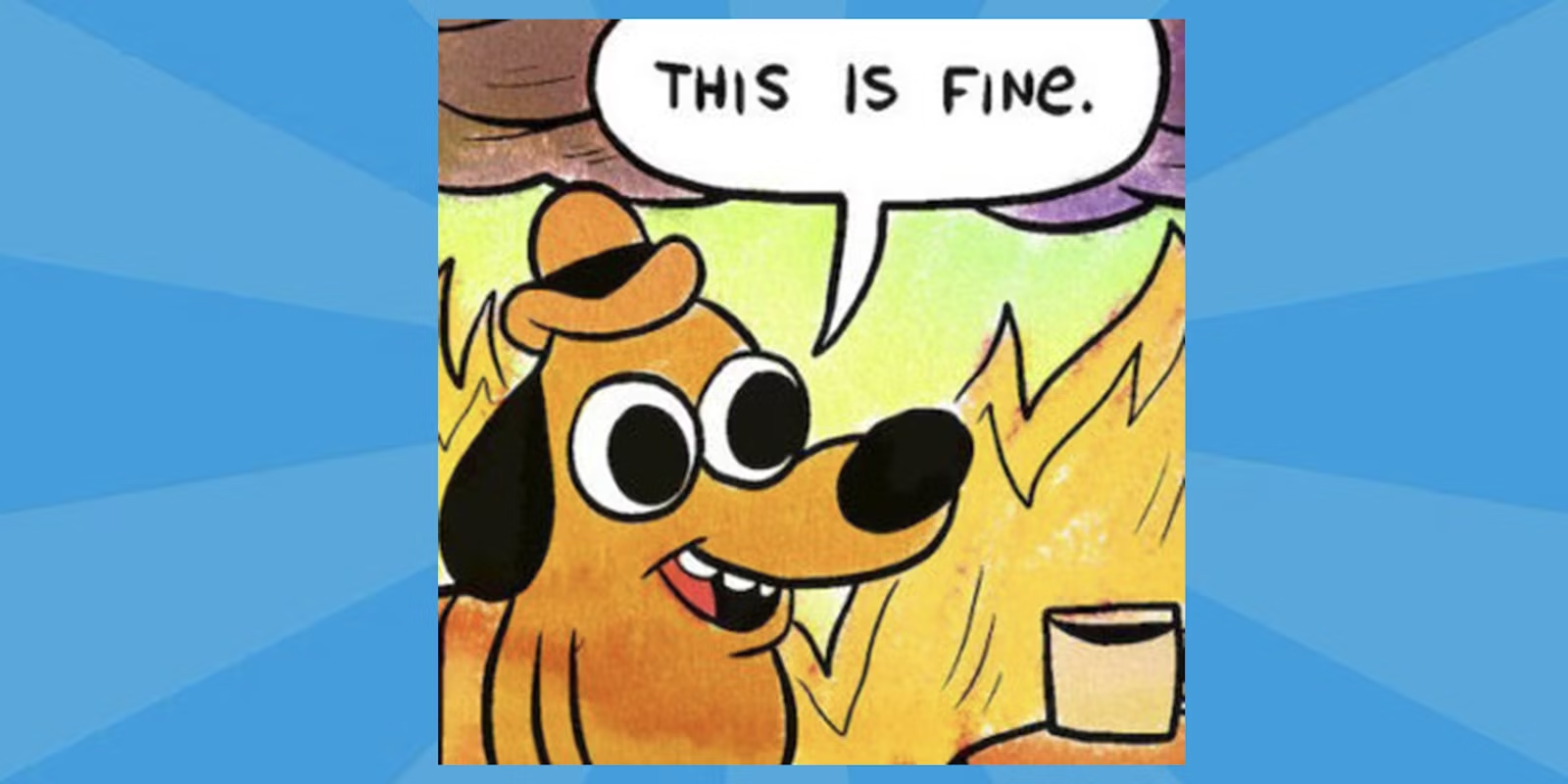
Too Many Tools
The tool landscape is overcrowded. Where once Active Directory was the go-to for authentication and authorization, we now have over ten alternatives, each with slight differences. This surplus of options is paralyzing.
The Paradox of Choice
When faced with too many options, our natural reaction is often to choose none. This phenomenon, known as “choice overload,” leaves engineers feeling overwhelmed and directionless.
Finding the Fix
Addressing this confusion and tool overload is challenging but feasible. Here’s a structured approach to mitigate these issues.
Reducing Confusion
Start by asking a single, crucial question: What’s the expected outcome?
Consider this scenario: Engineer A, excited by the Kubernetes hype, implements it without understanding its necessity, resulting in tech debt. Engineer B, however, asks about the expected outcome and then tailors a solution—Kubernetes or otherwise—to meet that goal. By focusing on outcomes, Engineer B avoids unnecessary complexity and chooses tools that align with specific needs.
Streamlining Tool Selection
Here’s a three-step approach to tackle the tool dilemma:
- Identify the Outcome: Begin by understanding the expected outcome, which helps in narrowing down the tool options.
- Research Thoroughly: Look into the tools that match your needs. Consult forums, read reviews, and gather opinions, but remember to take them with a grain of salt.
- Evaluate Rigorously: Test 2-3 tools extensively. Spend 3-4 days evaluating each one, paying attention to ease of installation, scalability, and usability.
- Strategic Tool Selection: Not a One-Size-Fits-All Deal.
My Perspective
As an experienced developer in multi-cloud enterprise architecture, I’ve seen firsthand how overwhelming cloud-native confusion and tool overload can be. I’ve found that the key to cutting through the noise is to focus on the essentials:
- What is the objective? Define clear goals for what you want to achieve.
- How do we reach that efficiently? Develop a straightforward, efficient plan to meet your goals.
- How do we keep operating and running the system, at best automatically? Aim for automation to ensure smooth, ongoing operations.
- How easy is it to change or add something? Ensure that your system is flexible enough to accommodate future changes.
It’s also crucial to get hands-on experience. Try out different solutions, build prototypes, and thoroughly test them before making a final decision. Avoid relying solely on procurement departments without knowing exactly what you need, as this can lead to unnecessary vendor conflicts and wasted resources.
What are your thoughts, cloud comrades? How do you cut through the noise and make sound decisions in the ever-evolving cloud-native landscape? Are there any tools or strategies you swear by? Let’s chat in the comments!